Golang中的经典设计模式
Golang中的经典设计模式
Golang是一个非常优秀的编程语言,它的设计理念和实现方式都非常值得我们学习和借鉴。在Golang中,设计模式也非常常见,今天,我们就来介绍一下Golang中的经典设计模式。
1. 单例模式
单例模式是一种常见的设计模式,它的作用是保证一个类只有一个实例,并提供一个全局访问点。在Golang中,单例模式可以通过sync.Once来实现。sync.Once是一个并发安全的类型,它可以保证在程序运行期间,某个函数只执行一次。
下面是一个简单的单例模式的示例:
package singletonimport ( "sync")type Singleton struct{}var instance *Singletonvar once sync.Oncefunc GetInstance() *Singleton { once.Do(func() { instance = &Singleton{} }) return instance}
2. 工厂方法模式
工厂方法模式是一种常见的创建型设计模式,它的作用是定义一个用于创建对象的接口,让子类决定实例化哪一个类。在Golang中,可以通过定义一个抽象工厂和具体工厂来实现工厂方法模式。
下面是一个简单的工厂方法模式的示例:
package factorytype Shape interface { Draw()}type Circle struct{}func (c *Circle) Draw() { fmt.Println("Draw a circle.")}type Rectangle struct{}func (r *Rectangle) Draw() { fmt.Println("Draw a rectangle.")}type ShapeFactory interface { CreateShape() Shape}type CircleFactory struct{}func (f *CircleFactory) CreateShape() Shape { return &Circle{}}type RectangleFactory struct{}func (f *RectangleFactory) CreateShape() Shape { return &Rectangle{}}
3. 建造者模式
建造者模式是一种常见的创建型设计模式,它的作用是将一个产品的构建过程抽象出来,使得这个过程可以独立于具体的产品类而存在。在Golang中,可以通过定义一个Builder接口和各种具体的Builder来实现建造者模式。
下面是一个简单的建造者模式的示例:
package buildertype Builder interface { BuildPartA() BuildPartB() BuildPartC() GetResult() interface{}}type Director struct { builder Builder}func (d *Director) SetBuilder(b Builder) { d.builder = b}func (d *Director) Construct() { d.builder.BuildPartA() d.builder.BuildPartB() d.builder.BuildPartC()}type Product struct { partA interface{} partB interface{} partC interface{}}func (p *Product) SetPartA(a interface{}) { p.partA = a}func (p *Product) SetPartB(b interface{}) { p.partB = b}func (p *Product) SetPartC(c interface{}) { p.partC = c}
以上就是Golang中的三种经典设计模式,它们分别是单例模式、工厂方法模式和建造者模式。当然,在实际的开发中,我们可能还会用到其他的设计模式,但是这三种模式足以涵盖大部分的情况。希望这篇文章对您有所帮助!

相关推荐HOT
更多>>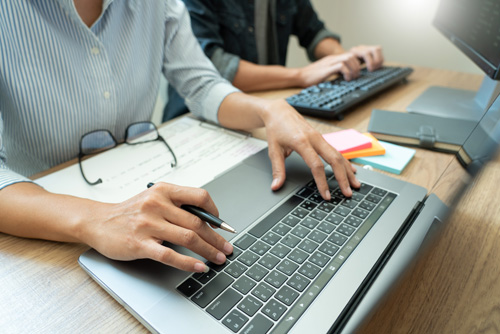
Golang并发模型深入解析
Golang并发模型深入解析在Go语言中,因其并发操作的高效性而受到广泛的关注。Go语言的核心哲学就是“不要通过共享内存来通信,而应该通过通信来...详情>>
2023-12-27 19:00:10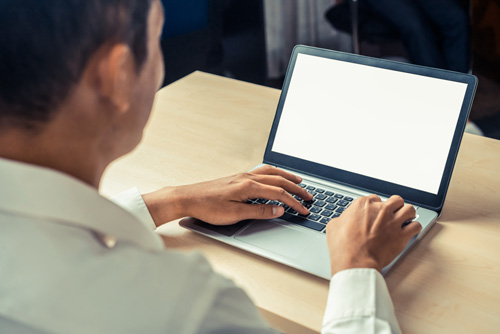
Go语言中的类型系统进阶指南
Go语言中的类型系统:进阶指南Go语言作为一门静态类型的编程语言,其类型系统是其最重要的特点之一,也是其广泛应用于微服务和云计算等领域的原...详情>>
2023-12-27 16:36:10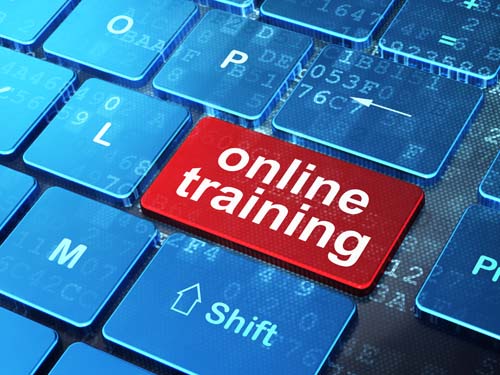
Golang中的错误处理优化
Golang中的错误处理优化错误处理在任何编程语言中都是非常重要的一部分。在Golang中,错误处理机制是内置的,通过返回值和错误对象来处理错误情...详情>>
2023-12-27 10:36:10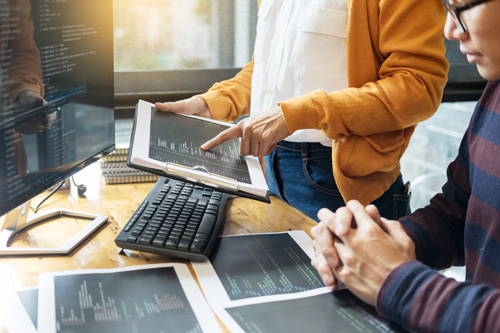
Golang中的反射与元编程
Golang 中的反射与元编程反射是计算机科学领域中的一项基础技术,它可以让程序在运行期间检查自身的结构和内容。Golang 的反射机制是非常强大的...详情>>
2023-12-26 23:48:09